forked from cerc-io/snowballtools-base
Part of [Service provider auctions for web deployments](https://www.notion.so/Service-provider-auctions-for-web-deployments-104a6b22d47280dbad51d28aa3a91d75) - Implement funtionality to pay for deployments by connecting wallet using `WalletConnect`  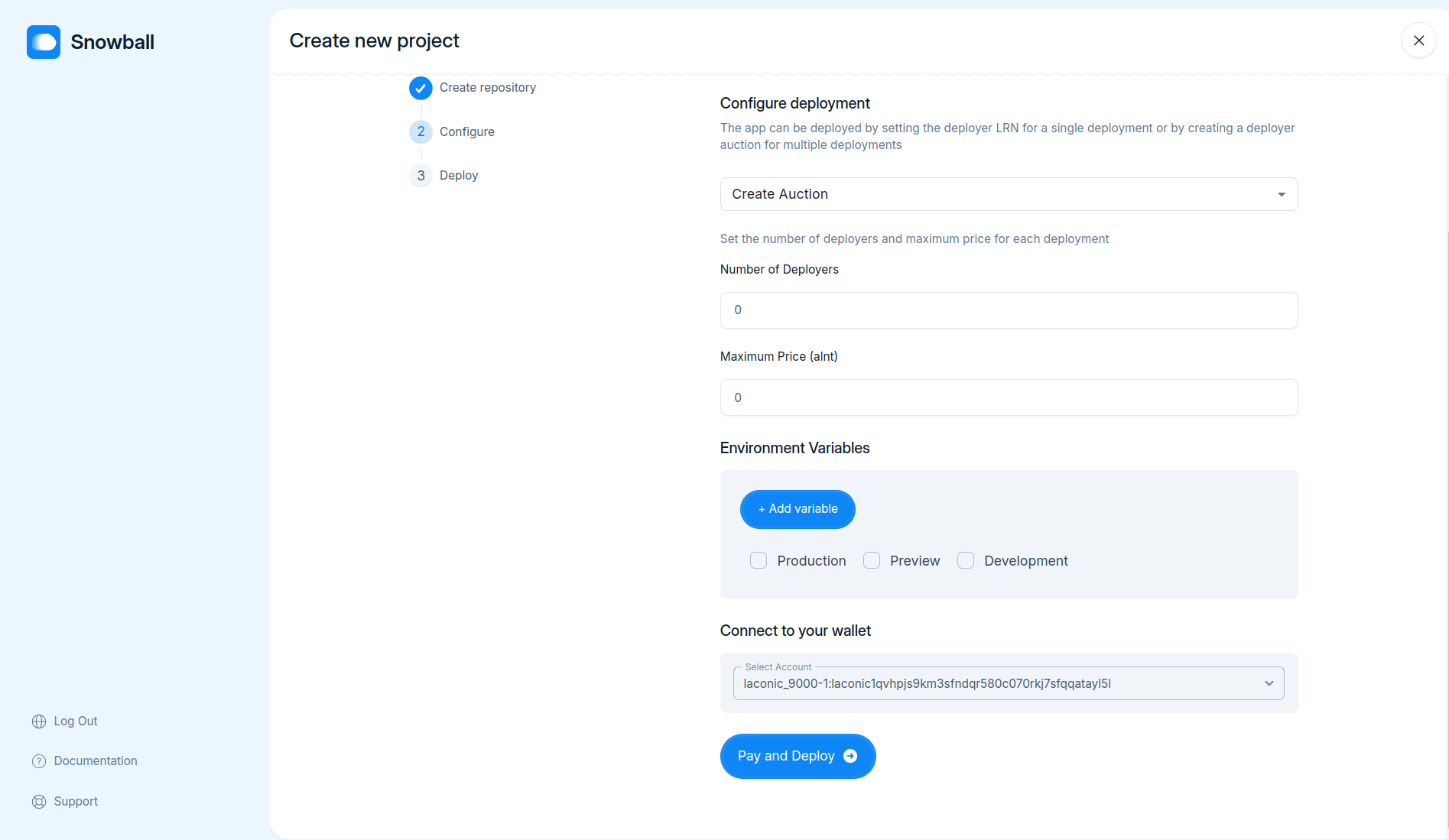 Co-authored-by: IshaVenikar <ishavenikar7@gmail.com> Co-authored-by: Shreerang Kale <shreerangkale@gmail.com> Reviewed-on: cerc-io/snowballtools-base#17
33 lines
978 B
TypeScript
33 lines
978 B
TypeScript
import { useEffect } from 'react';
|
|
import { useStopwatch } from 'react-timer-hook';
|
|
|
|
import FormatMillisecond, { FormatMilliSecondProps } from './FormatMilliSecond';
|
|
|
|
const setStopWatchOffset = (time: string) => {
|
|
const providedTime = new Date(time);
|
|
const currentTime = new Date();
|
|
const timeDifference = currentTime.getTime() - providedTime.getTime();
|
|
currentTime.setMilliseconds(currentTime.getMilliseconds() + timeDifference);
|
|
return currentTime;
|
|
};
|
|
|
|
interface StopwatchProps extends Omit<FormatMilliSecondProps, 'time'> {
|
|
offsetTimestamp: Date;
|
|
isPaused: boolean;
|
|
}
|
|
|
|
const Stopwatch = ({ offsetTimestamp, isPaused, ...props }: StopwatchProps) => {
|
|
const { totalSeconds, pause, start } = useStopwatch({
|
|
autoStart: true,
|
|
offsetTimestamp: offsetTimestamp,
|
|
});
|
|
|
|
useEffect(() => {
|
|
isPaused ? pause() : start();
|
|
}, [isPaused]);
|
|
|
|
return <FormatMillisecond time={totalSeconds * 1000} {...props} />;
|
|
};
|
|
|
|
export { Stopwatch, setStopWatchOffset };
|